2023-04-25 11:01:25 +00:00
|
|
|
/* eslint-disable @typescript-eslint/no-unused-vars */
|
2024-04-16 11:13:54 +00:00
|
|
|
import { LegacyMigrations, MigrationSequence } from '@tldraw/store'
|
|
|
|
import {
|
|
|
|
ShapeProps,
|
|
|
|
TLHandle,
|
|
|
|
TLShape,
|
|
|
|
TLShapePartial,
|
|
|
|
TLShapePropsMigrations,
|
|
|
|
TLUnknownShape,
|
|
|
|
} from '@tldraw/tlschema'
|
React-powered SVG exports (#3117)
## Migration path
1. If any of your shapes implement `toSvg` for exports, you'll need to
replace your implementation with a new version that returns JSX (it's a
react component) instead of manually constructing SVG DOM nodes
2. `editor.getSvg` is deprecated. It still works, but will be going away
in a future release. If you still need SVGs as DOM elements rather than
strings, use `new DOMParser().parseFromString(svgString,
'image/svg+xml').firstElementChild`
## The change in detail
At the moment, our SVG exports very carefully try to recreate the
visuals of our shapes by manually constructing SVG DOM nodes. On its own
this is really painful, but it also results in a lot of duplicated logic
between the `component` and `getSvg` methods of shape utils.
In #3020, we looked at using string concatenation & DOMParser to make
this a bit less painful. This works, but requires specifying namespaces
everywhere, is still pretty painful (no syntax highlighting or
formatting), and still results in all that duplicated logic.
I briefly experimented with creating my own version of the javascript
language that let you embed XML like syntax directly. I was going to
call it EXTREME JAVASCRIPT or XJS for short, but then I noticed that we
already wrote the whole of tldraw in this thing called react and a (imo
much worse named) version of the javascript xml thing already existed.
Given the entire library already depends on react, what would it look
like if we just used react directly for these exports? Turns out things
get a lot simpler! Take a look at lmk what you think
This diff was intended as a proof of concept, but is actually pretty
close to being landable. The main thing is that here, I've deliberately
leant into this being a big breaking change to see just how much code we
could delete (turns out: lots). We could if we wanted to make this
without making it a breaking change at all, but it would add back a lot
of complexity on our side and run a fair bit slower
---------
Co-authored-by: huppy-bot[bot] <128400622+huppy-bot[bot]@users.noreply.github.com>
2024-03-25 14:16:55 +00:00
|
|
|
import { ReactElement } from 'react'
|
2024-01-03 12:13:15 +00:00
|
|
|
import { Box } from '../../primitives/Box'
|
|
|
|
import { Vec } from '../../primitives/Vec'
|
`ShapeUtil.getGeometry`, selection rewrite (#1751)
This PR is a significant rewrite of our selection / hit testing logic.
It
- replaces our current geometric helpers (`getBounds`, `getOutline`,
`hitTestPoint`, and `hitTestLineSegment`) with a new geometry API
- moves our hit testing entirely to JS using geometry
- improves selection logic, especially around editing shapes, groups and
frames
- fixes many minor selection bugs (e.g. shapes behind frames)
- removes hit-testing DOM elements from ShapeFill etc.
- adds many new tests around selection
- adds new tests around selection
- makes several superficial changes to surface editor APIs
This PR is hard to evaluate. The `selection-omnibus` test suite is
intended to describe all of the selection behavior, however all existing
tests are also either here preserved and passing or (in a few cases
around editing shapes) are modified to reflect the new behavior.
## Geometry
All `ShapeUtils` implement `getGeometry`, which returns a single
geometry primitive (`Geometry2d`). For example:
```ts
class BoxyShapeUtil {
getGeometry(shape: BoxyShape) {
return new Rectangle2d({
width: shape.props.width,
height: shape.props.height,
isFilled: true,
margin: shape.props.strokeWidth
})
}
}
```
This geometric primitive is used for all bounds calculation, hit
testing, intersection with arrows, etc.
There are several geometric primitives that extend `Geometry2d`:
- `Arc2d`
- `Circle2d`
- `CubicBezier2d`
- `CubicSpline2d`
- `Edge2d`
- `Ellipse2d`
- `Group2d`
- `Polygon2d`
- `Rectangle2d`
- `Stadium2d`
For shapes that have more complicated geometric representations, such as
an arrow with a label, the `Group2d` can accept other primitives as its
children.
## Hit testing
Previously, we did all hit testing via events set on shapes and other
elements. In this PR, I've replaced those hit tests with our own
calculation for hit tests in JavaScript. This removed the need for many
DOM elements, such as hit test area borders and fills which only existed
to trigger pointer events.
## Selection
We now support selecting "hollow" shapes by clicking inside of them.
This involves a lot of new logic but it should work intuitively. See
`Editor.getShapeAtPoint` for the (thoroughly commented) implementation.

every sunset is actually the sun hiding in fear and respect of tldraw's
quality of interactions
This PR also fixes several bugs with scribble selection, in particular
around the shift key modifier.
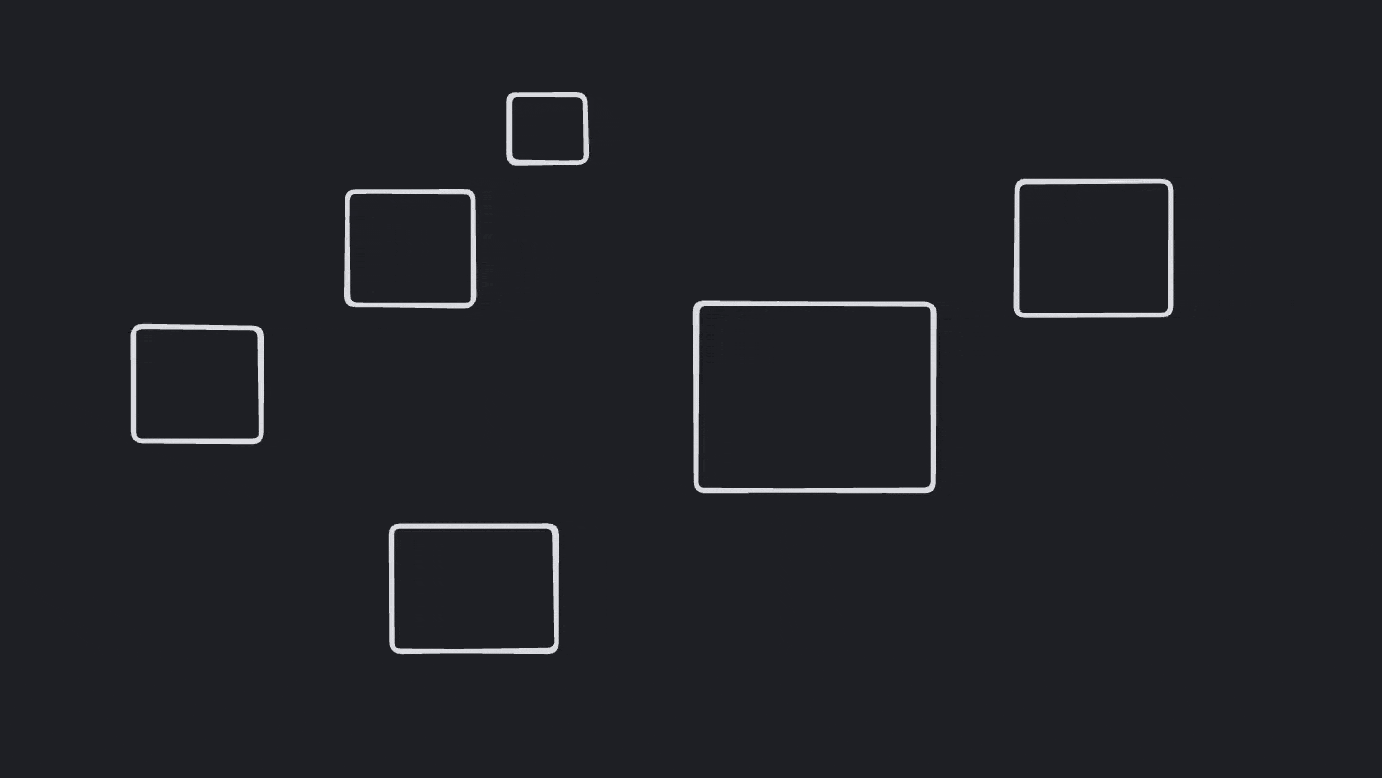
...as well as issues with labels and editing.
There are **over 100 new tests** for selection covering groups, frames,
brushing, scribbling, hovering, and editing. I'll add a few more before
I feel comfortable merging this PR.
## Arrow binding
Using the same "hollow shape" logic as selection, arrow binding is
significantly improved.

a thousand wise men could not improve on this
## Moving focus between editing shapes
Previously, this was handled in the `editing_shapes` state. This is
moved to `useEditableText`, and should generally be considered an
advanced implementation detail on a shape-by-shape basis. This addresses
a bug that I'd never noticed before, but which can be reproduced by
selecting an shape—but not focusing its input—while editing a different
shape. Previously, the new shape became the editing shape but its input
did not focus.
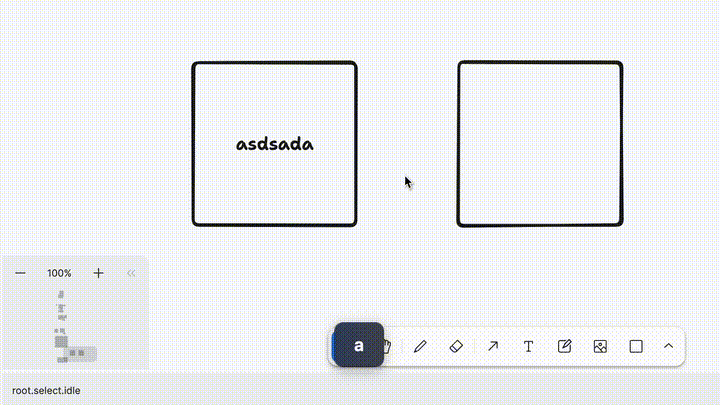
In this PR, you can select a shape by clicking on its edge or body, or
select its input to transfer editing / focus.

tldraw, glorious tldraw
### Change Type
- [x] `major` — Breaking change
### Test Plan
1. Erase shapes
2. Select shapes
3. Calculate their bounding boxes
- [ ] Unit Tests // todo
- [ ] End to end tests // todo
### Release Notes
- [editor] Remove `ShapeUtil.getBounds`, `ShapeUtil.getOutline`,
`ShapeUtil.hitTestPoint`, `ShapeUtil.hitTestLineSegment`
- [editor] Add `ShapeUtil.getGeometry`
- [editor] Add `Editor.getShapeGeometry`
2023-07-25 16:10:15 +00:00
|
|
|
import { Geometry2d } from '../../primitives/geometry/Geometry2d'
|
2023-06-02 15:21:45 +00:00
|
|
|
import type { Editor } from '../Editor'
|
[Snapping 3/5] Custom snapping API (#2793)
This diff adds an API for customising our existing snap types. These
are:
1. Bound snapping. When translating or resizing a shape, it'll snap to
certain key points on the bounds of particular shapes. Previously, these
were hard-coded to the corners and center of the bounding box of the
shape. Now, a shape can bring its own (e.g. a triangle may add snapping
for its 3 corners, and it's centroid rather than bounding box center.
2. Handle outline snapping. When dragging a handle, it'll snap to the
outline of other shapes geometry. Now, shapes can return different
geometry for this sort of snapping if they like.
Each of these is customised through a method on `ShapeUtil`:
`getBoundsSnapGeometry` and `getHandleSnapGeometry`. These return
interfaces describing the different geometry that can be snapped to in
both these cases. Currently, each returns an object with a single
property, but there are more types of snapping coming in follow-up PRs.
When reviewing this PR, start with the definitions of
`BoundsSnapGeometry` in `BoundsSnaps.ts` and `HandleSnapGeometry` in
`HandleSnaps.ts`
This doesn't add point snapping - i'll add that in a follow-up! It'll be
customisable with the `getHandleSnapGeometry` API.
Fixes TLD-2197
This PR is part of a series - please don't merge it until the things
before it have landed!
1. #2827
4. #2831
5. #2793 (you are here)
6. #2841
7. #2845
### Change Type
- [x] `minor` — New feature
[^1]: publishes a `patch` release, for devDependencies use `internal`
[^2]: will not publish a new version
### Test Plan
- [x] Unit Tests
### Release Notes
- Add `ShapeUtil.getSnapInfo` for customising shape snaps.
2024-02-15 15:10:04 +00:00
|
|
|
import { BoundsSnapGeometry } from '../managers/SnapManager/BoundsSnaps'
|
|
|
|
import { HandleSnapGeometry } from '../managers/SnapManager/HandleSnaps'
|
tldraw zero - package shuffle (#1710)
This PR moves code between our packages so that:
- @tldraw/editor is a “core” library with the engine and canvas but no
shapes, tools, or other things
- @tldraw/tldraw contains everything particular to the experience we’ve
built for tldraw
At first look, this might seem like a step away from customization and
configuration, however I believe it greatly increases the configuration
potential of the @tldraw/editor while also providing a more accurate
reflection of what configuration options actually exist for
@tldraw/tldraw.
## Library changes
@tldraw/editor re-exports its dependencies and @tldraw/tldraw re-exports
@tldraw/editor.
- users of @tldraw/editor WITHOUT @tldraw/tldraw should almost always
only import things from @tldraw/editor.
- users of @tldraw/tldraw should almost always only import things from
@tldraw/tldraw.
- @tldraw/polyfills is merged into @tldraw/editor
- @tldraw/indices is merged into @tldraw/editor
- @tldraw/primitives is merged mostly into @tldraw/editor, partially
into @tldraw/tldraw
- @tldraw/file-format is merged into @tldraw/tldraw
- @tldraw/ui is merged into @tldraw/tldraw
Many (many) utils and other code is moved from the editor to tldraw. For
example, embeds now are entirely an feature of @tldraw/tldraw. The only
big chunk of code left in core is related to arrow handling.
## API Changes
The editor can now be used without tldraw's assets. We load them in
@tldraw/tldraw instead, so feel free to use whatever fonts or images or
whatever that you like with the editor.
All tools and shapes (except for the `Group` shape) are moved to
@tldraw/tldraw. This includes the `select` tool.
You should use the editor with at least one tool, however, so you now
also need to send in an `initialState` prop to the Editor /
<TldrawEditor> component indicating which state the editor should begin
in.
The `components` prop now also accepts `SelectionForeground`.
The complex selection component that we use for tldraw is moved to
@tldraw/tldraw. The default component is quite basic but can easily be
replaced via the `components` prop. We pass down our tldraw-flavored
SelectionFg via `components`.
Likewise with the `Scribble` component: the `DefaultScribble` no longer
uses our freehand tech and is a simple path instead. We pass down the
tldraw-flavored scribble via `components`.
The `ExternalContentManager` (`Editor.externalContentManager`) is
removed and replaced with a mapping of types to handlers.
- Register new content handlers with
`Editor.registerExternalContentHandler`.
- Register new asset creation handlers (for files and URLs) with
`Editor.registerExternalAssetHandler`
### Change Type
- [x] `major` — Breaking change
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
- [@tldraw/editor] lots, wip
- [@tldraw/ui] gone, merged to tldraw/tldraw
- [@tldraw/polyfills] gone, merged to tldraw/editor
- [@tldraw/primitives] gone, merged to tldraw/editor / tldraw/tldraw
- [@tldraw/indices] gone, merged to tldraw/editor
- [@tldraw/file-format] gone, merged to tldraw/tldraw
---------
Co-authored-by: alex <alex@dytry.ch>
2023-07-17 21:22:34 +00:00
|
|
|
import { SvgExportContext } from '../types/SvgExportContext'
|
2023-04-25 11:01:25 +00:00
|
|
|
import { TLResizeHandle } from '../types/selection-types'
|
|
|
|
|
|
|
|
/** @public */
|
|
|
|
export interface TLShapeUtilConstructor<
|
|
|
|
T extends TLUnknownShape,
|
2024-01-15 12:33:15 +00:00
|
|
|
U extends ShapeUtil<T> = ShapeUtil<T>,
|
2023-04-25 11:01:25 +00:00
|
|
|
> {
|
tldraw zero - package shuffle (#1710)
This PR moves code between our packages so that:
- @tldraw/editor is a “core” library with the engine and canvas but no
shapes, tools, or other things
- @tldraw/tldraw contains everything particular to the experience we’ve
built for tldraw
At first look, this might seem like a step away from customization and
configuration, however I believe it greatly increases the configuration
potential of the @tldraw/editor while also providing a more accurate
reflection of what configuration options actually exist for
@tldraw/tldraw.
## Library changes
@tldraw/editor re-exports its dependencies and @tldraw/tldraw re-exports
@tldraw/editor.
- users of @tldraw/editor WITHOUT @tldraw/tldraw should almost always
only import things from @tldraw/editor.
- users of @tldraw/tldraw should almost always only import things from
@tldraw/tldraw.
- @tldraw/polyfills is merged into @tldraw/editor
- @tldraw/indices is merged into @tldraw/editor
- @tldraw/primitives is merged mostly into @tldraw/editor, partially
into @tldraw/tldraw
- @tldraw/file-format is merged into @tldraw/tldraw
- @tldraw/ui is merged into @tldraw/tldraw
Many (many) utils and other code is moved from the editor to tldraw. For
example, embeds now are entirely an feature of @tldraw/tldraw. The only
big chunk of code left in core is related to arrow handling.
## API Changes
The editor can now be used without tldraw's assets. We load them in
@tldraw/tldraw instead, so feel free to use whatever fonts or images or
whatever that you like with the editor.
All tools and shapes (except for the `Group` shape) are moved to
@tldraw/tldraw. This includes the `select` tool.
You should use the editor with at least one tool, however, so you now
also need to send in an `initialState` prop to the Editor /
<TldrawEditor> component indicating which state the editor should begin
in.
The `components` prop now also accepts `SelectionForeground`.
The complex selection component that we use for tldraw is moved to
@tldraw/tldraw. The default component is quite basic but can easily be
replaced via the `components` prop. We pass down our tldraw-flavored
SelectionFg via `components`.
Likewise with the `Scribble` component: the `DefaultScribble` no longer
uses our freehand tech and is a simple path instead. We pass down the
tldraw-flavored scribble via `components`.
The `ExternalContentManager` (`Editor.externalContentManager`) is
removed and replaced with a mapping of types to handlers.
- Register new content handlers with
`Editor.registerExternalContentHandler`.
- Register new asset creation handlers (for files and URLs) with
`Editor.registerExternalAssetHandler`
### Change Type
- [x] `major` — Breaking change
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
- [@tldraw/editor] lots, wip
- [@tldraw/ui] gone, merged to tldraw/tldraw
- [@tldraw/polyfills] gone, merged to tldraw/editor
- [@tldraw/primitives] gone, merged to tldraw/editor / tldraw/tldraw
- [@tldraw/indices] gone, merged to tldraw/editor
- [@tldraw/file-format] gone, merged to tldraw/tldraw
---------
Co-authored-by: alex <alex@dytry.ch>
2023-07-17 21:22:34 +00:00
|
|
|
new (editor: Editor): U
|
[refactor] User-facing APIs (#1478)
This PR updates our user-facing APIs for the Tldraw and TldrawEditor
components, as well as the Editor (App). It mainly incorporates surface
changes from #1450 without any changes to validators or migrators,
incorporating feedback / discussion with @SomeHats and @ds300.
Here we:
- remove the TldrawEditorConfig
- bring back a loose version of shape definitions
- make a separation between "core" shapes and "default" shapes
- do not allow custom shapes, migrators or validators to overwrite core
shapes
- but _do_ allow new shapes
## `<Tldraw>` component
In this PR, the `Tldraw` component wraps both the `TldrawEditor`
component and our `TldrawUi` component. It accepts a union of props for
both components. Previously, this component also added local syncing via
a `useLocalSyncClient` hook call, however that has been pushed down to
the `TldrawEditor` component.
## `<TldrawEditor>` component
The `TldrawEditor` component now more neatly wraps up the different ways
that the editor can be configured.
## The store prop (`TldrawEditorProps.store`)
There are three main ways for the `TldrawEditor` component to be run:
1. with an externally defined store
2. with an externally defined syncing store (local or remote)
3. with an internally defined store
4. with an internally defined locally syncing store
The `store` prop allows for these configurations.
If the `store` prop is defined, it may be defined either as a `TLStore`
or as a `SyncedStore`. If the store is a `TLStore`, then the Editor will
assume that the store is ready to go; if it is defined as a SyncedStore,
then the component will display the loading / error screens as needed,
or the final editor once the store's status is "synced".
When the store is left undefined, then the `TldrawEditor` will create
its own internal store using the optional `instanceId`, `initialData`,
or `shapes` props to define the store / store schema.
If the `persistenceKey` prop is left undefined, then the store will not
be synced. If the `persistenceKey` is defined, then the store will be
synced locally. In the future, we may also here accept the API key /
roomId / etc for creating a remotely synced store.
The `SyncedStore` type has been expanded to also include types used for
remote syncing, e.g. with `ConnectionStatus`.
## Tools
By default, the App has two "baked-in" tools: the select tool and the
zoom tool. These cannot (for now) be replaced or removed. The default
tools are used by default, but may be replaced by other tools if
provided.
## Shapes
By default, the App has a set of "core" shapes:
- group
- embed
- bookmark
- image
- video
- text
That cannot by overwritten because they're created by the app at
different moments, such as when double clicking on the canvas or via a
copy and paste event. In follow up PRs, we'll split these out so that
users can replace parts of the code where these shapes are created.
### Change Type
- [x] `major` — Breaking Change
### Test Plan
- [x] Unit Tests
2023-06-01 15:47:34 +00:00
|
|
|
type: T['type']
|
tldraw zero - package shuffle (#1710)
This PR moves code between our packages so that:
- @tldraw/editor is a “core” library with the engine and canvas but no
shapes, tools, or other things
- @tldraw/tldraw contains everything particular to the experience we’ve
built for tldraw
At first look, this might seem like a step away from customization and
configuration, however I believe it greatly increases the configuration
potential of the @tldraw/editor while also providing a more accurate
reflection of what configuration options actually exist for
@tldraw/tldraw.
## Library changes
@tldraw/editor re-exports its dependencies and @tldraw/tldraw re-exports
@tldraw/editor.
- users of @tldraw/editor WITHOUT @tldraw/tldraw should almost always
only import things from @tldraw/editor.
- users of @tldraw/tldraw should almost always only import things from
@tldraw/tldraw.
- @tldraw/polyfills is merged into @tldraw/editor
- @tldraw/indices is merged into @tldraw/editor
- @tldraw/primitives is merged mostly into @tldraw/editor, partially
into @tldraw/tldraw
- @tldraw/file-format is merged into @tldraw/tldraw
- @tldraw/ui is merged into @tldraw/tldraw
Many (many) utils and other code is moved from the editor to tldraw. For
example, embeds now are entirely an feature of @tldraw/tldraw. The only
big chunk of code left in core is related to arrow handling.
## API Changes
The editor can now be used without tldraw's assets. We load them in
@tldraw/tldraw instead, so feel free to use whatever fonts or images or
whatever that you like with the editor.
All tools and shapes (except for the `Group` shape) are moved to
@tldraw/tldraw. This includes the `select` tool.
You should use the editor with at least one tool, however, so you now
also need to send in an `initialState` prop to the Editor /
<TldrawEditor> component indicating which state the editor should begin
in.
The `components` prop now also accepts `SelectionForeground`.
The complex selection component that we use for tldraw is moved to
@tldraw/tldraw. The default component is quite basic but can easily be
replaced via the `components` prop. We pass down our tldraw-flavored
SelectionFg via `components`.
Likewise with the `Scribble` component: the `DefaultScribble` no longer
uses our freehand tech and is a simple path instead. We pass down the
tldraw-flavored scribble via `components`.
The `ExternalContentManager` (`Editor.externalContentManager`) is
removed and replaced with a mapping of types to handlers.
- Register new content handlers with
`Editor.registerExternalContentHandler`.
- Register new asset creation handlers (for files and URLs) with
`Editor.registerExternalAssetHandler`
### Change Type
- [x] `major` — Breaking change
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
- [@tldraw/editor] lots, wip
- [@tldraw/ui] gone, merged to tldraw/tldraw
- [@tldraw/polyfills] gone, merged to tldraw/editor
- [@tldraw/primitives] gone, merged to tldraw/editor / tldraw/tldraw
- [@tldraw/indices] gone, merged to tldraw/editor
- [@tldraw/file-format] gone, merged to tldraw/tldraw
---------
Co-authored-by: alex <alex@dytry.ch>
2023-07-17 21:22:34 +00:00
|
|
|
props?: ShapeProps<T>
|
2024-04-16 11:13:54 +00:00
|
|
|
migrations?: LegacyMigrations | TLShapePropsMigrations | MigrationSequence
|
2023-04-25 11:01:25 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/** @public */
|
|
|
|
export type TLShapeUtilFlag<T> = (shape: T) => boolean
|
|
|
|
|
2023-06-24 13:46:04 +00:00
|
|
|
/** @public */
|
|
|
|
export interface TLShapeUtilCanvasSvgDef {
|
|
|
|
key: string
|
|
|
|
component: React.ComponentType
|
|
|
|
}
|
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-16 10:33:47 +00:00
|
|
|
export abstract class ShapeUtil<Shape extends TLUnknownShape = TLUnknownShape> {
|
tldraw zero - package shuffle (#1710)
This PR moves code between our packages so that:
- @tldraw/editor is a “core” library with the engine and canvas but no
shapes, tools, or other things
- @tldraw/tldraw contains everything particular to the experience we’ve
built for tldraw
At first look, this might seem like a step away from customization and
configuration, however I believe it greatly increases the configuration
potential of the @tldraw/editor while also providing a more accurate
reflection of what configuration options actually exist for
@tldraw/tldraw.
## Library changes
@tldraw/editor re-exports its dependencies and @tldraw/tldraw re-exports
@tldraw/editor.
- users of @tldraw/editor WITHOUT @tldraw/tldraw should almost always
only import things from @tldraw/editor.
- users of @tldraw/tldraw should almost always only import things from
@tldraw/tldraw.
- @tldraw/polyfills is merged into @tldraw/editor
- @tldraw/indices is merged into @tldraw/editor
- @tldraw/primitives is merged mostly into @tldraw/editor, partially
into @tldraw/tldraw
- @tldraw/file-format is merged into @tldraw/tldraw
- @tldraw/ui is merged into @tldraw/tldraw
Many (many) utils and other code is moved from the editor to tldraw. For
example, embeds now are entirely an feature of @tldraw/tldraw. The only
big chunk of code left in core is related to arrow handling.
## API Changes
The editor can now be used without tldraw's assets. We load them in
@tldraw/tldraw instead, so feel free to use whatever fonts or images or
whatever that you like with the editor.
All tools and shapes (except for the `Group` shape) are moved to
@tldraw/tldraw. This includes the `select` tool.
You should use the editor with at least one tool, however, so you now
also need to send in an `initialState` prop to the Editor /
<TldrawEditor> component indicating which state the editor should begin
in.
The `components` prop now also accepts `SelectionForeground`.
The complex selection component that we use for tldraw is moved to
@tldraw/tldraw. The default component is quite basic but can easily be
replaced via the `components` prop. We pass down our tldraw-flavored
SelectionFg via `components`.
Likewise with the `Scribble` component: the `DefaultScribble` no longer
uses our freehand tech and is a simple path instead. We pass down the
tldraw-flavored scribble via `components`.
The `ExternalContentManager` (`Editor.externalContentManager`) is
removed and replaced with a mapping of types to handlers.
- Register new content handlers with
`Editor.registerExternalContentHandler`.
- Register new asset creation handlers (for files and URLs) with
`Editor.registerExternalAssetHandler`
### Change Type
- [x] `major` — Breaking change
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
- [@tldraw/editor] lots, wip
- [@tldraw/ui] gone, merged to tldraw/tldraw
- [@tldraw/polyfills] gone, merged to tldraw/editor
- [@tldraw/primitives] gone, merged to tldraw/editor / tldraw/tldraw
- [@tldraw/indices] gone, merged to tldraw/editor
- [@tldraw/file-format] gone, merged to tldraw/tldraw
---------
Co-authored-by: alex <alex@dytry.ch>
2023-07-17 21:22:34 +00:00
|
|
|
constructor(public editor: Editor) {}
|
|
|
|
static props?: ShapeProps<TLUnknownShape>
|
2024-04-16 11:13:54 +00:00
|
|
|
static migrations?: LegacyMigrations | TLShapePropsMigrations
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
2023-06-05 14:49:44 +00:00
|
|
|
* The type of the shape util, which should match the shape's type.
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-05 14:49:44 +00:00
|
|
|
static type: string
|
2023-04-25 11:01:25 +00:00
|
|
|
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
/**
|
|
|
|
* Get the default props for a shape.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
abstract getDefaultProps(): Shape['props']
|
|
|
|
|
`ShapeUtil.getGeometry`, selection rewrite (#1751)
This PR is a significant rewrite of our selection / hit testing logic.
It
- replaces our current geometric helpers (`getBounds`, `getOutline`,
`hitTestPoint`, and `hitTestLineSegment`) with a new geometry API
- moves our hit testing entirely to JS using geometry
- improves selection logic, especially around editing shapes, groups and
frames
- fixes many minor selection bugs (e.g. shapes behind frames)
- removes hit-testing DOM elements from ShapeFill etc.
- adds many new tests around selection
- adds new tests around selection
- makes several superficial changes to surface editor APIs
This PR is hard to evaluate. The `selection-omnibus` test suite is
intended to describe all of the selection behavior, however all existing
tests are also either here preserved and passing or (in a few cases
around editing shapes) are modified to reflect the new behavior.
## Geometry
All `ShapeUtils` implement `getGeometry`, which returns a single
geometry primitive (`Geometry2d`). For example:
```ts
class BoxyShapeUtil {
getGeometry(shape: BoxyShape) {
return new Rectangle2d({
width: shape.props.width,
height: shape.props.height,
isFilled: true,
margin: shape.props.strokeWidth
})
}
}
```
This geometric primitive is used for all bounds calculation, hit
testing, intersection with arrows, etc.
There are several geometric primitives that extend `Geometry2d`:
- `Arc2d`
- `Circle2d`
- `CubicBezier2d`
- `CubicSpline2d`
- `Edge2d`
- `Ellipse2d`
- `Group2d`
- `Polygon2d`
- `Rectangle2d`
- `Stadium2d`
For shapes that have more complicated geometric representations, such as
an arrow with a label, the `Group2d` can accept other primitives as its
children.
## Hit testing
Previously, we did all hit testing via events set on shapes and other
elements. In this PR, I've replaced those hit tests with our own
calculation for hit tests in JavaScript. This removed the need for many
DOM elements, such as hit test area borders and fills which only existed
to trigger pointer events.
## Selection
We now support selecting "hollow" shapes by clicking inside of them.
This involves a lot of new logic but it should work intuitively. See
`Editor.getShapeAtPoint` for the (thoroughly commented) implementation.

every sunset is actually the sun hiding in fear and respect of tldraw's
quality of interactions
This PR also fixes several bugs with scribble selection, in particular
around the shift key modifier.
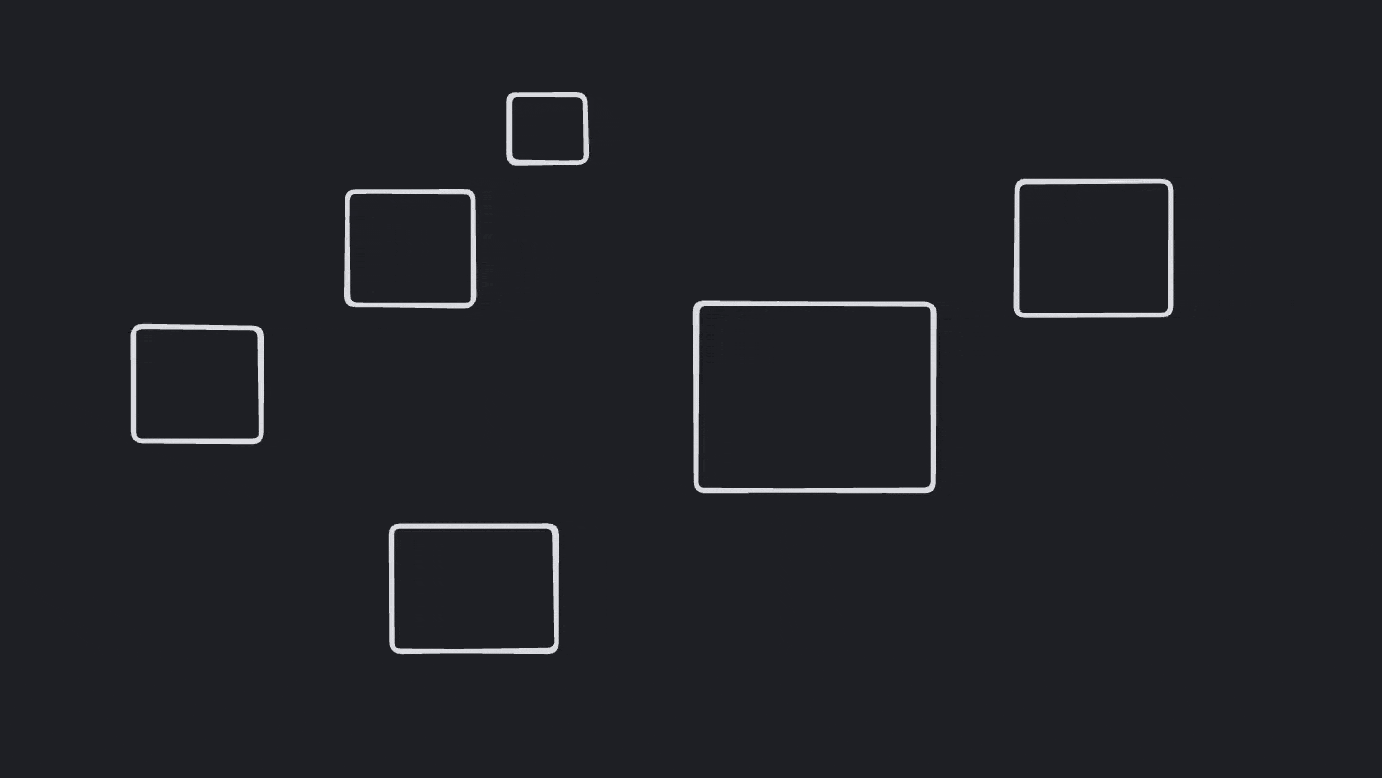
...as well as issues with labels and editing.
There are **over 100 new tests** for selection covering groups, frames,
brushing, scribbling, hovering, and editing. I'll add a few more before
I feel comfortable merging this PR.
## Arrow binding
Using the same "hollow shape" logic as selection, arrow binding is
significantly improved.

a thousand wise men could not improve on this
## Moving focus between editing shapes
Previously, this was handled in the `editing_shapes` state. This is
moved to `useEditableText`, and should generally be considered an
advanced implementation detail on a shape-by-shape basis. This addresses
a bug that I'd never noticed before, but which can be reproduced by
selecting an shape—but not focusing its input—while editing a different
shape. Previously, the new shape became the editing shape but its input
did not focus.
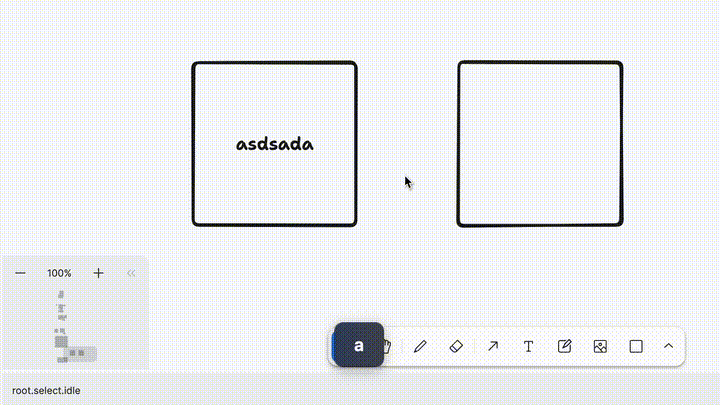
In this PR, you can select a shape by clicking on its edge or body, or
select its input to transfer editing / focus.

tldraw, glorious tldraw
### Change Type
- [x] `major` — Breaking change
### Test Plan
1. Erase shapes
2. Select shapes
3. Calculate their bounding boxes
- [ ] Unit Tests // todo
- [ ] End to end tests // todo
### Release Notes
- [editor] Remove `ShapeUtil.getBounds`, `ShapeUtil.getOutline`,
`ShapeUtil.hitTestPoint`, `ShapeUtil.hitTestLineSegment`
- [editor] Add `ShapeUtil.getGeometry`
- [editor] Add `Editor.getShapeGeometry`
2023-07-25 16:10:15 +00:00
|
|
|
/**
|
|
|
|
* Get the shape's geometry.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
abstract getGeometry(shape: Shape): Geometry2d
|
|
|
|
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
/**
|
|
|
|
* Get a JSX element for the shape (as an HTML element).
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
abstract component(shape: Shape): any
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Get JSX describing the shape's indicator (as an SVG element).
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
abstract indicator(shape: Shape): any
|
|
|
|
|
2023-06-15 14:36:46 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape can be snapped to by another shape.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canSnap: TLShapeUtilFlag<Shape> = () => true
|
2023-06-15 14:36:46 +00:00
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape can be scrolled while editing.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canScroll: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Whether the shape can be bound to by an arrow.
|
|
|
|
*
|
|
|
|
* @param _otherShape - The other shape attempting to bind to this shape.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canBind = <K>(_shape: Shape, _otherShape?: K) => true
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Whether the shape can be double clicked to edit.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canEdit: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Whether the shape can be resized.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canResize: TLShapeUtilFlag<Shape> = () => true
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2023-10-03 11:03:01 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape can be edited in read-only mode.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
canEditInReadOnly: TLShapeUtilFlag<Shape> = () => false
|
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape can be cropped.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canCrop: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
* Does this shape provide a background for its children? If this is true,
|
|
|
|
* then any children with a `renderBackground` method will have their
|
|
|
|
* backgrounds rendered _above_ this shape. Otherwise, the children's
|
|
|
|
* backgrounds will be rendered above either the next ancestor that provides
|
|
|
|
* a background, or the canvas background.
|
2023-04-25 11:01:25 +00:00
|
|
|
*
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
* @internal
|
2023-04-25 11:01:25 +00:00
|
|
|
*/
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
providesBackgroundForChildren(shape: Shape): boolean {
|
|
|
|
return false
|
2023-04-25 11:01:25 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Whether the shape should hide its resize handles when selected.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
hideResizeHandles: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
2024-01-15 13:41:48 +00:00
|
|
|
* Whether the shape should hide its rotation handles when selected.
|
2023-04-25 11:01:25 +00:00
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
hideRotateHandle: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2023-07-26 15:32:33 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape should hide its selection bounds background when selected.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
hideSelectionBoundsBg: TLShapeUtilFlag<Shape> = () => false
|
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/**
|
|
|
|
* Whether the shape should hide its selection bounds foreground when selected.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
hideSelectionBoundsFg: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Whether the shape's aspect ratio is locked.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
isAspectRatioLocked: TLShapeUtilFlag<Shape> = () => false
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2023-06-01 15:22:47 +00:00
|
|
|
/**
|
|
|
|
* Get a JSX element for the shape (as an HTML element) to be rendered as part of the canvas background - behind any other shape content.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @internal
|
|
|
|
*/
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
backgroundComponent?(shape: Shape): any
|
2023-06-01 15:22:47 +00:00
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/**
|
|
|
|
* Get an array of handle models for the shape. This is an optional method.
|
|
|
|
*
|
|
|
|
* @example
|
|
|
|
*
|
|
|
|
* ```ts
|
|
|
|
* util.getHandles?.(myShape)
|
|
|
|
* ```
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @public
|
|
|
|
*/
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
getHandles?(shape: Shape): TLHandle[]
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Get whether the shape can receive children of a given type.
|
|
|
|
*
|
|
|
|
* @param type - The shape type.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canReceiveNewChildrenOfType(shape: Shape, type: TLShape['type']) {
|
2023-04-25 11:01:25 +00:00
|
|
|
return false
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Get whether the shape can receive children of a given type.
|
|
|
|
*
|
|
|
|
* @param shape - The shape type.
|
|
|
|
* @param shapes - The shapes that are being dropped.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
canDropShapes(shape: Shape, shapes: TLShape[]) {
|
2023-04-25 11:01:25 +00:00
|
|
|
return false
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Get the shape as an SVG object.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
2023-06-24 13:46:04 +00:00
|
|
|
* @param ctx - The export context for the SVG - used for adding e.g. \<def\>s
|
2023-04-25 11:01:25 +00:00
|
|
|
* @returns An SVG element.
|
|
|
|
* @public
|
|
|
|
*/
|
React-powered SVG exports (#3117)
## Migration path
1. If any of your shapes implement `toSvg` for exports, you'll need to
replace your implementation with a new version that returns JSX (it's a
react component) instead of manually constructing SVG DOM nodes
2. `editor.getSvg` is deprecated. It still works, but will be going away
in a future release. If you still need SVGs as DOM elements rather than
strings, use `new DOMParser().parseFromString(svgString,
'image/svg+xml').firstElementChild`
## The change in detail
At the moment, our SVG exports very carefully try to recreate the
visuals of our shapes by manually constructing SVG DOM nodes. On its own
this is really painful, but it also results in a lot of duplicated logic
between the `component` and `getSvg` methods of shape utils.
In #3020, we looked at using string concatenation & DOMParser to make
this a bit less painful. This works, but requires specifying namespaces
everywhere, is still pretty painful (no syntax highlighting or
formatting), and still results in all that duplicated logic.
I briefly experimented with creating my own version of the javascript
language that let you embed XML like syntax directly. I was going to
call it EXTREME JAVASCRIPT or XJS for short, but then I noticed that we
already wrote the whole of tldraw in this thing called react and a (imo
much worse named) version of the javascript xml thing already existed.
Given the entire library already depends on react, what would it look
like if we just used react directly for these exports? Turns out things
get a lot simpler! Take a look at lmk what you think
This diff was intended as a proof of concept, but is actually pretty
close to being landable. The main thing is that here, I've deliberately
leant into this being a big breaking change to see just how much code we
could delete (turns out: lots). We could if we wanted to make this
without making it a breaking change at all, but it would add back a lot
of complexity on our side and run a fair bit slower
---------
Co-authored-by: huppy-bot[bot] <128400622+huppy-bot[bot]@users.noreply.github.com>
2024-03-25 14:16:55 +00:00
|
|
|
toSvg?(shape: Shape, ctx: SvgExportContext): ReactElement | null | Promise<ReactElement | null>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2023-06-01 15:22:47 +00:00
|
|
|
/**
|
|
|
|
* Get the shape's background layer as an SVG object.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
2023-06-24 13:46:04 +00:00
|
|
|
* @param ctx - ctx - The export context for the SVG - used for adding e.g. \<def\>s
|
2023-06-01 15:22:47 +00:00
|
|
|
* @returns An SVG element.
|
|
|
|
* @public
|
|
|
|
*/
|
React-powered SVG exports (#3117)
## Migration path
1. If any of your shapes implement `toSvg` for exports, you'll need to
replace your implementation with a new version that returns JSX (it's a
react component) instead of manually constructing SVG DOM nodes
2. `editor.getSvg` is deprecated. It still works, but will be going away
in a future release. If you still need SVGs as DOM elements rather than
strings, use `new DOMParser().parseFromString(svgString,
'image/svg+xml').firstElementChild`
## The change in detail
At the moment, our SVG exports very carefully try to recreate the
visuals of our shapes by manually constructing SVG DOM nodes. On its own
this is really painful, but it also results in a lot of duplicated logic
between the `component` and `getSvg` methods of shape utils.
In #3020, we looked at using string concatenation & DOMParser to make
this a bit less painful. This works, but requires specifying namespaces
everywhere, is still pretty painful (no syntax highlighting or
formatting), and still results in all that duplicated logic.
I briefly experimented with creating my own version of the javascript
language that let you embed XML like syntax directly. I was going to
call it EXTREME JAVASCRIPT or XJS for short, but then I noticed that we
already wrote the whole of tldraw in this thing called react and a (imo
much worse named) version of the javascript xml thing already existed.
Given the entire library already depends on react, what would it look
like if we just used react directly for these exports? Turns out things
get a lot simpler! Take a look at lmk what you think
This diff was intended as a proof of concept, but is actually pretty
close to being landable. The main thing is that here, I've deliberately
leant into this being a big breaking change to see just how much code we
could delete (turns out: lots). We could if we wanted to make this
without making it a breaking change at all, but it would add back a lot
of complexity on our side and run a fair bit slower
---------
Co-authored-by: huppy-bot[bot] <128400622+huppy-bot[bot]@users.noreply.github.com>
2024-03-25 14:16:55 +00:00
|
|
|
toBackgroundSvg?(
|
|
|
|
shape: Shape,
|
|
|
|
ctx: SvgExportContext
|
|
|
|
): ReactElement | null | Promise<ReactElement | null>
|
2023-06-01 15:22:47 +00:00
|
|
|
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
/** @internal */
|
|
|
|
expandSelectionOutlinePx(shape: Shape): number {
|
|
|
|
return 0
|
|
|
|
}
|
|
|
|
|
2023-06-24 13:46:04 +00:00
|
|
|
/**
|
|
|
|
* Return elements to be added to the \<defs\> section of the canvases SVG context. This can be
|
|
|
|
* used to define SVG content (e.g. patterns & masks) that can be referred to by ID from svg
|
|
|
|
* elements returned by `component`.
|
|
|
|
*
|
|
|
|
* Each def should have a unique `key`. If multiple defs from different shapes all have the same
|
|
|
|
* key, only one will be used.
|
|
|
|
*/
|
|
|
|
getCanvasSvgDefs(): TLShapeUtilCanvasSvgDef[] {
|
|
|
|
return []
|
|
|
|
}
|
|
|
|
|
[Snapping 3/5] Custom snapping API (#2793)
This diff adds an API for customising our existing snap types. These
are:
1. Bound snapping. When translating or resizing a shape, it'll snap to
certain key points on the bounds of particular shapes. Previously, these
were hard-coded to the corners and center of the bounding box of the
shape. Now, a shape can bring its own (e.g. a triangle may add snapping
for its 3 corners, and it's centroid rather than bounding box center.
2. Handle outline snapping. When dragging a handle, it'll snap to the
outline of other shapes geometry. Now, shapes can return different
geometry for this sort of snapping if they like.
Each of these is customised through a method on `ShapeUtil`:
`getBoundsSnapGeometry` and `getHandleSnapGeometry`. These return
interfaces describing the different geometry that can be snapped to in
both these cases. Currently, each returns an object with a single
property, but there are more types of snapping coming in follow-up PRs.
When reviewing this PR, start with the definitions of
`BoundsSnapGeometry` in `BoundsSnaps.ts` and `HandleSnapGeometry` in
`HandleSnaps.ts`
This doesn't add point snapping - i'll add that in a follow-up! It'll be
customisable with the `getHandleSnapGeometry` API.
Fixes TLD-2197
This PR is part of a series - please don't merge it until the things
before it have landed!
1. #2827
4. #2831
5. #2793 (you are here)
6. #2841
7. #2845
### Change Type
- [x] `minor` — New feature
[^1]: publishes a `patch` release, for devDependencies use `internal`
[^2]: will not publish a new version
### Test Plan
- [x] Unit Tests
### Release Notes
- Add `ShapeUtil.getSnapInfo` for customising shape snaps.
2024-02-15 15:10:04 +00:00
|
|
|
/**
|
|
|
|
* Get the geometry to use when snapping to this this shape in translate/resize operations. See
|
|
|
|
* {@link BoundsSnapGeometry} for details.
|
|
|
|
*/
|
|
|
|
getBoundsSnapGeometry(shape: Shape): BoundsSnapGeometry {
|
|
|
|
return {}
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Get the geometry to use when snapping handles to this shape. See {@link HandleSnapGeometry}
|
|
|
|
* for details.
|
|
|
|
*/
|
|
|
|
getHandleSnapGeometry(shape: Shape): HandleSnapGeometry {
|
|
|
|
return {}
|
|
|
|
}
|
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
// Events
|
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called just before a shape is created. This method provides a last chance to modify
|
|
|
|
* the created shape.
|
|
|
|
*
|
|
|
|
* @example
|
|
|
|
*
|
|
|
|
* ```ts
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
* onBeforeCreate = (next) => {
|
|
|
|
* return { ...next, x: next.x + 1 }
|
2023-04-25 11:01:25 +00:00
|
|
|
* }
|
|
|
|
* ```
|
|
|
|
*
|
|
|
|
* @param next - The next shape.
|
|
|
|
* @returns The next shape or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onBeforeCreate?: TLOnBeforeCreateHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called just before a shape is updated. This method provides a last chance to modify
|
|
|
|
* the updated shape.
|
|
|
|
*
|
|
|
|
* @example
|
|
|
|
*
|
|
|
|
* ```ts
|
|
|
|
* onBeforeUpdate = (prev, next) => {
|
|
|
|
* if (prev.x === next.x) {
|
|
|
|
* return { ...next, x: next.x + 1 }
|
|
|
|
* }
|
|
|
|
* }
|
|
|
|
* ```
|
|
|
|
*
|
|
|
|
* @param prev - The previous shape.
|
|
|
|
* @param next - The next shape.
|
|
|
|
* @returns The next shape or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onBeforeUpdate?: TLOnBeforeUpdateHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
|
|
|
* A callback called when some other shapes are dragged over this one.
|
|
|
|
*
|
|
|
|
* @example
|
|
|
|
*
|
|
|
|
* ```ts
|
|
|
|
* onDragShapesOver = (shape, shapes) => {
|
2024-04-14 18:40:02 +00:00
|
|
|
* this.editor.reparentShapes(shapes, shape.id)
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
* }
|
|
|
|
* ```
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @param shapes - The shapes that are being dragged over this one.
|
|
|
|
* @public
|
|
|
|
*/
|
2024-04-14 18:40:02 +00:00
|
|
|
onDragShapesOver?: TLOnDragHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when some other shapes are dragged out of this one.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @param shapes - The shapes that are being dragged out.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onDragShapesOut?: TLOnDragHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when some other shapes are dropped over this one.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @param shapes - The shapes that are being dropped over this one.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onDropShapesOver?: TLOnDragHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
|
|
|
* A callback called when a shape starts being resized.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onResizeStart?: TLOnResizeStartHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape changes from a resize.
|
|
|
|
*
|
|
|
|
* @param shape - The shape at the start of the resize.
|
|
|
|
* @param info - Info about the resize.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onResize?: TLOnResizeHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape finishes resizing.
|
|
|
|
*
|
|
|
|
* @param initial - The shape at the start of the resize.
|
|
|
|
* @param current - The current shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onResizeEnd?: TLOnResizeEndHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
|
|
|
* A callback called when a shape starts being translated.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onTranslateStart?: TLOnTranslateStartHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape changes from a translation.
|
|
|
|
*
|
|
|
|
* @param initial - The shape at the start of the translation.
|
|
|
|
* @param current - The current shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onTranslate?: TLOnTranslateHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape finishes translating.
|
|
|
|
*
|
|
|
|
* @param initial - The shape at the start of the translation.
|
|
|
|
* @param current - The current shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onTranslateEnd?: TLOnTranslateEndHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2024-01-24 10:19:20 +00:00
|
|
|
/**
|
|
|
|
* A callback called when a shape's handle changes.
|
|
|
|
*
|
|
|
|
* @param shape - The current shape.
|
|
|
|
* @param info - An object containing the handle and whether the handle is 'precise' or not.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
onHandleDrag?: TLOnHandleDragHandler<Shape>
|
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
|
|
|
* A callback called when a shape starts being rotated.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onRotateStart?: TLOnRotateStartHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape changes from a rotation.
|
|
|
|
*
|
|
|
|
* @param initial - The shape at the start of the rotation.
|
|
|
|
* @param current - The current shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onRotate?: TLOnRotateHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/**
|
|
|
|
* A callback called when a shape finishes rotating.
|
|
|
|
*
|
|
|
|
* @param initial - The shape at the start of the rotation.
|
|
|
|
* @param current - The current shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onRotateEnd?: TLOnRotateEndHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Not currently used.
|
|
|
|
*
|
|
|
|
* @internal
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onBindingChange?: TLOnBindingChangeHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape's children change.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns An array of shape updates, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onChildrenChange?: TLOnChildrenChangeHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape's handle is double clicked.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @param handle - The handle that is double-clicked.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onDoubleClickHandle?: TLOnDoubleClickHandleHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape's edge is double clicked.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onDoubleClickEdge?: TLOnDoubleClickHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape is double clicked.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onDoubleClick?: TLOnDoubleClickHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape is clicked.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @returns A change to apply to the shape, or void.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onClick?: TLOnClickHandler<Shape>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* A callback called when a shape finishes being editing.
|
|
|
|
*
|
|
|
|
* @param shape - The shape.
|
|
|
|
* @public
|
|
|
|
*/
|
2023-06-16 10:33:47 +00:00
|
|
|
onEditEnd?: TLOnEditEndHandler<Shape>
|
2023-04-25 11:01:25 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnBeforeCreateHandler<T extends TLShape> = (next: T) => T | void
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnBeforeUpdateHandler<T extends TLShape> = (prev: T, next: T) => T | void
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnTranslateStartHandler<T extends TLShape> = TLEventStartHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnTranslateHandler<T extends TLShape> = TLEventChangeHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnTranslateEndHandler<T extends TLShape> = TLEventChangeHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnRotateStartHandler<T extends TLShape> = TLEventStartHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnRotateHandler<T extends TLShape> = TLEventChangeHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnRotateEndHandler<T extends TLShape> = TLEventChangeHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/**
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
* The type of resize.
|
|
|
|
*
|
|
|
|
* 'scale_shape' - The shape is being scaled, usually as part of a larger selection.
|
|
|
|
*
|
2023-04-25 11:01:25 +00:00
|
|
|
* 'resize_bounds' - The user is directly manipulating an individual shape's bounds using a resize
|
|
|
|
* handle. It is up to shape util implementers to decide how they want to handle the two
|
|
|
|
* situations.
|
|
|
|
*
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
export type TLResizeMode = 'scale_shape' | 'resize_bounds'
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
|
|
|
/**
|
|
|
|
* Info about a resize.
|
|
|
|
* @param newPoint - The new local position of the shape.
|
|
|
|
* @param handle - The handle being dragged.
|
|
|
|
* @param mode - The type of resize.
|
|
|
|
* @param scaleX - The scale in the x-axis.
|
|
|
|
* @param scaleY - The scale in the y-axis.
|
|
|
|
* @param initialBounds - The bounds of the shape at the start of the resize.
|
|
|
|
* @param initialShape - The shape at the start of the resize.
|
|
|
|
* @public
|
|
|
|
*/
|
|
|
|
export type TLResizeInfo<T extends TLShape> = {
|
2024-01-03 12:13:15 +00:00
|
|
|
newPoint: Vec
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
handle: TLResizeHandle
|
|
|
|
mode: TLResizeMode
|
|
|
|
scaleX: number
|
|
|
|
scaleY: number
|
2024-01-03 12:13:15 +00:00
|
|
|
initialBounds: Box
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
initialShape: T
|
|
|
|
}
|
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnResizeHandler<T extends TLShape> = (
|
2023-04-25 11:01:25 +00:00
|
|
|
shape: T,
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
info: TLResizeInfo<T>
|
`ShapeUtil` refactor, `Editor` cleanup (#1611)
This PR improves the ergonomics of `ShapeUtil` classes.
### Cached methods
First, I've remove the cached methods (such as `bounds`) from the
`ShapeUtil` class and lifted this to the `Editor` class.
Previously, calling `ShapeUtil.getBounds` would return the un-cached
bounds of a shape, while calling `ShapeUtil.bounds` would return the
cached bounds of a shape. We also had `Editor.getBounds`, which would
call `ShapeUtil.bounds`. It was confusing. The cached methods like
`outline` were also marked with "please don't override", which suggested
the architecture was just wrong.
The only weirdness from this is that utils sometimes reach out to the
editor for cached versions of data rather than calling their own cached
methods. It's still an easier story to tell than what we had before.
### More defaults
We now have three and only three `abstract` methods for a `ShapeUtil`:
- `getDefaultProps` (renamed from `defaultProps`)
- `getBounds`,
- `component`
- `indicator`
Previously, we also had `getCenter` as an abstract method, though this
was usually just the middle of the bounds anyway.
### Editing bounds
This PR removes the concept of editingBounds. The viewport will no
longer animate to editing shapes.
### Active area manager
This PR also removes the active area manager, which was not being used
in the way we expected it to be.
### Dpr manager
This PR removes the dpr manager and uses a hook instead to update it
from React. This is one less runtime browser dependency in the app, one
less thing to document.
### Moving things around
This PR also continues to try to organize related methods and properties
in the editor.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [editor] renames `defaultProps` to `getDefaultProps`
- [editor] removes `outline`, `outlineSegments`, `handles`, `bounds`
- [editor] renames `renderBackground` to `backgroundComponent`
2023-06-19 14:01:18 +00:00
|
|
|
) => Omit<TLShapePartial<T>, 'id' | 'type'> | undefined | void
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnResizeStartHandler<T extends TLShape> = TLEventStartHandler<T>
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnResizeEndHandler<T extends TLShape> = TLEventChangeHandler<T>
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/* -------------------- Dragging -------------------- */
|
|
|
|
|
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnDragHandler<T extends TLShape, R = void> = (shape: T, shapes: TLShape[]) => R
|
2023-04-25 11:01:25 +00:00
|
|
|
|
add docs for TLShapeUtil (#1215)
This PR adds docs for the methods in the TLShapeUtil class.
I think that it's a good page to have docs on, as it shows people what's
possible with the custom shape API.
Currently, our docs are not showing `@param` info for lots of methods,
including the ones added in this PR.
I'll do fix for that in a follow-up PR, so that it's easier to review.
---
Note: Moving forward, we probably want to consider **_where_** these
docs are shown, and how we achieve that.
For example, do we put the docs for these methods in:
* The docs page for the `TLShapeUtil` class?
* The docs pages for the handler types, eg:
[`OnResizeHandler`](http://localhost:3000/gen/editor/OnResizeHandler-type)?
* Both?
Right now, I opted for putting them in the the TLShapeUtil class, as it
keeps them all in one place, and it's what we already do for some
others.
We should consider both - what works best for the docs? and what works
best for code editors?
---
This PR also includes a fix to our pre-commit step that @SomeHats did.
2023-05-05 14:05:25 +00:00
|
|
|
/** @internal */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnBindingChangeHandler<T extends TLShape> = (shape: T) => TLShapePartial<T> | void
|
2023-04-25 11:01:25 +00:00
|
|
|
|
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnChildrenChangeHandler<T extends TLShape> = (shape: T) => TLShapePartial[] | void
|
2023-04-25 11:01:25 +00:00
|
|
|
|
2024-01-24 10:19:20 +00:00
|
|
|
/** @public */
|
|
|
|
export type TLOnHandleDragHandler<T extends TLShape> = (
|
2023-04-25 11:01:25 +00:00
|
|
|
shape: T,
|
|
|
|
info: {
|
|
|
|
handle: TLHandle
|
|
|
|
isPrecise: boolean
|
2023-10-27 13:33:50 +00:00
|
|
|
initial?: T | undefined
|
2023-04-25 11:01:25 +00:00
|
|
|
}
|
|
|
|
) => TLShapePartial<T> | void
|
|
|
|
|
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnClickHandler<T extends TLShape> = (shape: T) => TLShapePartial<T> | void
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnEditEndHandler<T extends TLShape> = (shape: T) => void
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnDoubleClickHandler<T extends TLShape> = (shape: T) => TLShapePartial<T> | void
|
2023-04-25 11:01:25 +00:00
|
|
|
/** @public */
|
2023-06-04 10:38:53 +00:00
|
|
|
export type TLOnDoubleClickHandleHandler<T extends TLShape> = (
|
2023-04-25 11:01:25 +00:00
|
|
|
shape: T,
|
|
|
|
handle: TLHandle
|
|
|
|
) => TLShapePartial<T> | void
|
|
|
|
|
2023-06-04 10:38:53 +00:00
|
|
|
type TLEventStartHandler<T extends TLShape> = (shape: T) => TLShapePartial<T> | void
|
|
|
|
type TLEventChangeHandler<T extends TLShape> = (initial: T, current: T) => TLShapePartial<T> | void
|